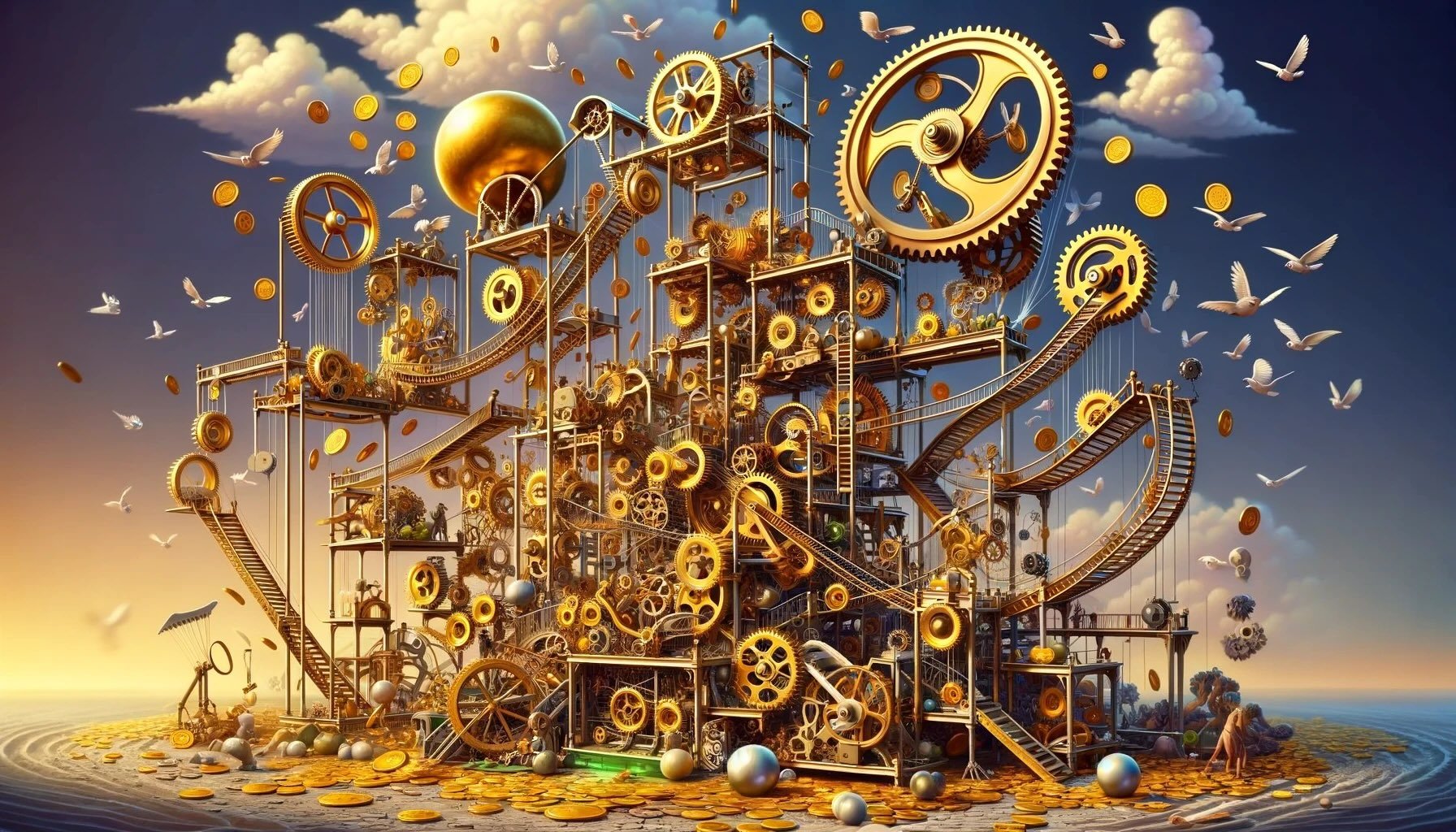
Uniswap v4 and Automated Market Makers (AMMs)
Uniswap v4 marks the latest iteration in the evolution of the Uniswap protocol, aiming to refine the decentralised trading experience on the Ethereum blockchain. This version introduces several technical enhancements, most notably the integration of customisable hooks, which allow developers to modify and extend the protocol's functionality to accommodate a wide range of applications.
Moving Plists Between Macs
I use a few apps where I would like to synchronise preferences between 2 MacBooks.
Unfortunately, there seems to be no way to do this automatically, but a manual workaround is to export the plist to a folder that can sync over Dropbox/iCloud, etc., then import it on the 2nd Mac.
As an example to copy Amethyst settings from a source to a target mac:
Javascript imports and exports
Functions, objects and primitive values can be exported from a module. A module can have many named exports and one default export, ES6 favours a default export per module with additional helper functions exposed as named exports. Exports can be marked ‘in-line’ or separately where the practise generally is to export at the end of the file. Exports can be aliased with ‘as’ and writing export default baz is equivalent to export { baz as default }.
What is Lambda Calculus
Lambda Calculus is a simple and very sparse notation for representing and applying functions.
The format is:λx.xWhere
λ signifies it is a Lambda function
The x before the period represents the input to the function
The function body follows the period
So λx.x is the equivalent of:
function(x) {return x}